Here we will discuss how we can implement a particular post type specific search functionality in WordPress.
Before implementing post type specific search, we first need to know what post type is in WordPress.
Table of Contents
Post type in WordPress
In WordPress, there are many different types of content. These types of content are usually defined as post types.
In the database, all of the post types are stored in wp_posts table.
In this table, there is a column named post_type on the value of which we can differentiate different post types.
When we create a new page by clicking Pages -> Add New link from the admin section menu, the new record is saved in wp_posts table and post_type is saved as page.
Similarly when we create a new post by clicking the Posts -> Add New link, the new record is saved in the wp_posts table and the post_type is saved as post.
In WordPress, there are several default post types like post, page, attachment, etc.
We can know more details about the post types in WordPress at: https://developer.wordpress.org/themes/basics/post-types/
In addition to the default post types, we can create our own post types in WordPress.
For example, on an e-commerce website, we may need to create a product post type.
We can learn more about creating custom post types at: https://developer.wordpress.org/plugins/post-types/registering-custom-post-types/
Now we will discuss implementing post type specific search in WordPress.
Post type specific search in WordPress
We discussed about implementation of search functionality in WordPress at: https://webtechbased.com/how-to-implement-search-functionality-in-wordpress/.
There we learned about 4 ways to implement search functionality –
process 1. Using WordPress function get_search_form(),
process 2. Using the WordPress Widget Panel,
Process 3. Creating a custom search form,
Process 4. Writing a custom function and hooking that function to the get_search_form.
Post type specific search for custom HTML search form
In case of 3 or 4 no processes, we are writing the HTML of the search form by ourselves. Now if we write hidden input type HTML code like below, then we can easily perform post type specific search.
<input type="hidden" value="post" name="post_type" id="post_type" />
So the final HTML of the custom search form would be:
<form action="<?php echo home_url( '/' );?>" method="get">
<div>
<label class="screen-reader-text" for="search">Search</label>
<input type="text" name="s" id="search" value="<?php the_search_query(); ?>" />
<input type="hidden" value="post" name="post_type" id="post_type" />
<input type="submit" id="customsearchsubmit" value="Search" />
<div>
</form>
Pre condition of custom post type specific search
But in the case of custom post type, we need to keep some things in mind. if we create a custom post type using the register_post_type WordPress function we can pass array or string of arguments for registering a post type.
register_post_type( string $post_type, array|string $args = array() )
Here we see the second parameter is $args. In these arguments, we need to look at public and exclude_from_search options.
The default value of public is false. If we want to use it publicly (like displaying to the front end) we can set it to true.
The default settings of exclude_from_search is inherited from the public.
The default value of exclude_from_search is the opposite value of public. This value determines whether the contents of this post type would appear in the search results or not.
This value of exclude_from_search should be false to get the search result of this post type in the front end.
$args = array(
// ...
'exclude_from_search' => true,
// ...
);
Post type specific search for WordPress features
Now we will discuss if we have implemented search functionality using process 1: get_search_form() or procees 2: Using the WordPress Widget Panel.
If we write the following code in the functions.pp file then we can set a specific post type for the search functionality. Here we have set post as post type for search.
// Search only posts for search
add_filter('pre_get_posts','SearchFilter');
function SearchFilter($query) {
if ($query->is_search) {
$query->set('post_type', 'post');
}
return $query;
}
Browser output for post type specific search
Now if we click on the search button of the search form at the front end after adding any value like ‘test’ to the input field then we will be redirected to http://<SITEURL>/?s=test in the browser according to the search form action.
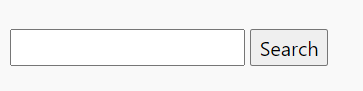
In this case, we can see only ‘post’ post type data in the search result.
In this way we can implement post type specific search even where we have implemented search functionality by creating a custom HTML form and in that case if we are using the above code we will not need to use the following HTML code in search form:
<input type="hidden" value="product" name="post_type" id="post_type" />