We can implement search functionality in WordPress in many ways. We will discuss these one by one.
Table of Contents
Processes to implement search functionality
Process 1: Use WordPress function:
We can generate a search form using the get_search_form() function in any of our theme files (for example, header.php).
<?php echo get_search_form();?>
We can get more details about this function at this URL: https://developer.wordpress.org/reference/functions/get_search_form/
Process 2: Use the WordPress Widget Panel:
Through this, we can easily implement the search bar but for that, we need to register the widget area first.
To register the widget area we can write the following code in the theme’s functions.php file.
function site_widgets_init() {
register_sidebar(
array(
'name' => 'Sidebar',
'id' => 'sidebar-1',
'description' => 'Add widgets here to appear in Sidebar',
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
)
);
}
add_action( 'widgets_init', 'site_widgets_init' );
After registering the widget area, we need to display the widget in the theme. We can write the following code in a theme file (for example sidebar.php file).
<?php if ( is_active_sidebar( 'sidebar-1' ) ) : ?>
<?php dynamic_sidebar( 'sidebar-1' ); ?>
<?php
endif;
?>
After that, we need to log in to the backend/admin of the WordPress site.
Then we have to navigate to Appearance -> Widgets from our WordPress dashboard.
So, as we can see in the following images, we need to look for a widget called Search and select this.
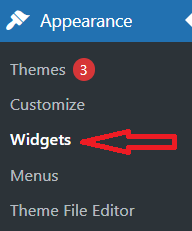
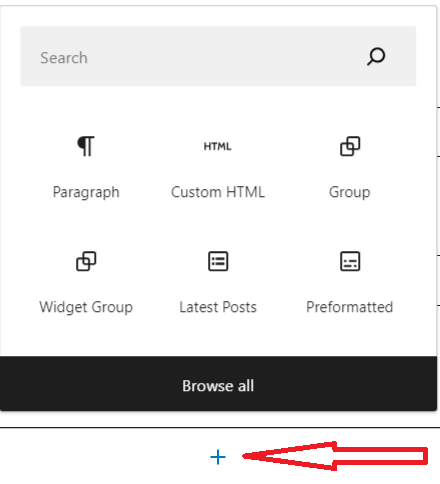
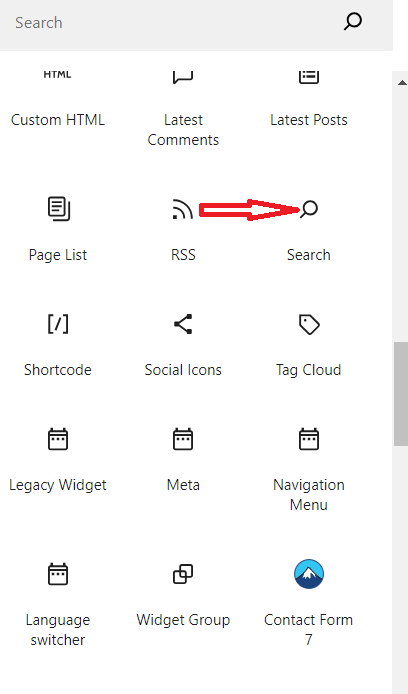
Now if we select the search widget here then we see the search widget added as below::

Then we have to save the page by clicking on the update button and the search form will be displayed on the front end.
Process 3: Create a custom search form
We can create a custom search form in any of file (like, sidebar.php) of the currently active theme.
<form action="<?php echo home_url( '/' );?>" method="get">
<div>
<label class="screen-reader-text" for="search">Search</label>
<input type="text" name="s" id="search" value="<?php the_search_query(); ?>" />
<input type="submit" id="customsearchsubmit" value="Search" />
<div>
</form>
Process 4: Create a custom function and use that function to action hook.
We can write a custom function (in current theme’s functions.php file) and use that function to the get_search_form action hook to generate a custom search form.
function custom_search_form( $form ) {
$form = '<form role="search" method="get" id="searchform" class="searchform" action="' . home_url( '/' ) . '" >
<div><label class="screen-reader-text" for="s">' . __( 'Search for:' ) . '</label>
<input type="text" value="' . get_search_query() . '" name="s" id="s" />
<input type="submit" id="hooksearchsubmit" value="'. esc_attr__( 'Search' ) .'" />
</div>
</form>';
return $form;
}
add_filter( 'get_search_form', 'custom_search_form' );
Browser output
If we follow any of the processes mentioned above, we can see the search form at the front end.
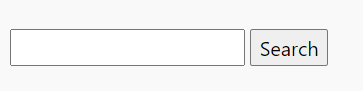
If we click on the search button by adding any value like ‘test’ to the input field then we will be redirected to http://<SITEURL>/?s=test
in the browser according to the search form action.
1 thought on “How to implement search functionality in WordPress?”
Comments are closed.